- Failure is Feedback
- Posts
- ✅ Quick Tip: Generating Files with Cy.WriteFile
✅ Quick Tip: Generating Files with Cy.WriteFile
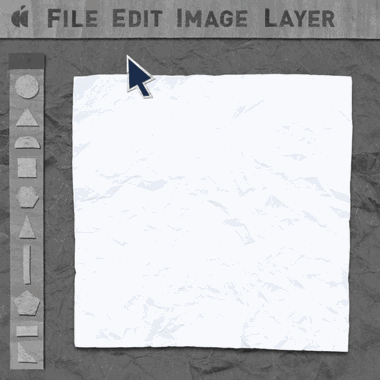
If we’re going to talk about files, first take a moment to remember Clippy.

Now that I’ve dated myself, let’s discuss how to test file imports using Cypress.
When googling file imports, there is plenty of great documentation to sift through. While I was excited to try out Sam E Lawrence’s implementation of Drag and Drop, I realized, in this use case, it wouldn’t fit my needs. Keep scrolling if you’d like to read my journey of testing file imports.
TL;DR
Create a Command that handles file imports
To use Cypress’ .selectFile, use Filip Hric’s suggestion:
cy.get("[data-cy=upload-file]") .selectFile('cypress/fixtures/logo.png', { action: 'drag-drop' })
What the test looks like when everything is in place
// import-test.cy.tscy.writeImportFile("cypress/support/fixtures/test-file.xlsx"); cy.get("[data-cy=upload-file]").selectFile( "cypress/support/fixtures/test-file.xlsx", { action: "drag-drop" }, );
Add both files to .gitignore so that you are not inserting files into the test suite’s repository
.gitignore# cypress fixture filesui_packages/cypress-shared/support/fixtures/csv-test.csvui_packages/cypress-shared/support/fixtures/xlsx-test.xlsx
File imports weren’t exactly new to me, but I hadn’t created a file import test in a while. As my brain dumps everything it views as unnecessary, I had to start from scratch.
Reaching out to the Cypress Discord Community, I was pointed to Filip Hric’s post about Uploading to a Dropzone. Perfect for my use case, I created a file with static user information and dropped it in the fixtures folder in Cypress. (Side note: you can literally drop the file into the fixtures folder in VS Code. For some reason, I thought you were required to add the file some other way.)
Then I ran the test using Filip’s suggestion:
cy.get("[data-cy=upload-file]") .selectFile('cypress/fixtures/logo.png', { action: 'drag-drop' })
🎆 Success! Import test passed!
But, I didn’t want to stop there. I wanted to have a generated file with unique user information every time.
Googling more, I found Oka Mahardika’s excellent post about generating CSV’s. I’ll add that while he recommended importing cypress-fs-extra into the project, I did not find that necessary.
Using his suggestion as a guide, I took the test one step further by creating a command. I formatted it to be able to generate CSV or XSLX files:
// commands.tsimport { faker } from '@faker-js/faker';interface Chainable { /** * generate an import file * @example cy.writeImportFile(file) */ writeImportFile(file: string): Chainable<JQuery<HTMLElement>>; }// values for writing an import file// user oneconst name = faker.person.fullName();const email = faker.internet.email();const number = faker.phone.number()// user twoconst name1 = faker.person.fullName();const email1 = faker.internet.email();const number1 = faker.phone.number()const data = [ ["fullName", "email", "mobileNumber"], [name, email, number], [name1, email1, number1],];Cypress.Commands.add("writeImportFile", (file) => { const content = data .map((row) => { return row.join(","); }) .join("\n"); cy.writeFile(file, content);});
Let’s refresh 🚰
This line generates the file:
cy.writeImportFile("cypress/support/fixtures/test-file.xlsx");
Now we’ll implement the drag-and-drop to read from the generated file:
cy.get("[data-cy=upload-file]").selectFile( "cypress/support/fixtures/test-file.xlsx", { action: "drag-drop" }, );
Put it all together and…
cy.writeImportFile("cypress/support/fixtures/test-file.xlsx");cy.get("[data-cy=upload-file]").selectFile( "cypress/support/fixtures/test-file.xlsx", { action: "drag-drop" }, );
Important note: The file is created as an XLSX or CSV based on how you denote what the file type is. The string you insert into the cy.writeImportFile command tells Cypress to generate a file with this particular name, the file type, and where you want it stored.
"cypress/support/fixtures/test-file.xlsx"
The final step was to add the file identifiers to .gitignore so both files would not be added to the test suite when pushed to the repository.
.gitignore# cypress fixture filescypress/support/fixtures/test-file.csvcypress/support/fixtures/test-file.xlsx
Pure magic 🪄
And, I know that even this can be improved on. At this point, I stopped and declared done being better than perfect.
I hope this helps you when you need to test imports with Cypress.
Till next time…

Written with 🏛️School of Athens l Immersive Experience (4K) Teravibe playing in the background
Reply