- Failure is Feedback
- Posts
- ✅ Quick Tip: .as() if! Accessing Aliases in beforeEach/afterEach Hooks
✅ Quick Tip: .as() if! Accessing Aliases in beforeEach/afterEach Hooks

Early in my automation journey, I learned it’s a better practice to use API calls to set up your tests instead of using the UI each time. This creates faster and more reliable tests. Most days, using the API is fine. It’s worth it.
But.
I found myself stepping into what is known as Callback Hell. This is when you need to use and access multiple aliases to build and verify your test’s outcome. Here’s one discussion on Stackoverflow I’ve referenced a few times.
Now I’m thinking of all the code I need to refactor once this post is published. I digress…
Recently, I found myself falling into callback hell again. The tests I wrote looked messy, not DRY, and not friendly from a troubleshooting perspective. I thought about how to structure the tests and then it struck me…

What if I set the aliases in the beforeEach and afterEach hooks?
Buuuuuuuuuuut…
Finding good documentation on how to set aliases in a beforeEach/afterEach was sparse. It took a little digging and throwing some spaghetti at the wall but I got there.
TL;DR: Use .as() + .then(function () {}) to access Aliases
beforeEach(() => { cy.createUser(name, email); cy.get("@id").as("contactId");});afterEach(() => { cy.get("@contactId").then(function () { cy.deleteContact(this.contactId); });});describe("Experimenting with aliases", () => { it("A User is created", () => { cy.get("@contactId").then(function () { cy.api({ headers, auth, method: "GET", url: "/url/used/to/test/for/an/existing/user",, }).then((response) => { // Expecting the response status code to be 200 expect(response.status).to.eq(200); expect(response.body).contain("users", `${this.contactId}`); }); }); });});// use .as() in combination with .then(function () {})// access the this.alias in the .it() block (even with multiple tests) and in the afterEach()

My first bit of the puzzle was the use of .as()
beforeEach(() => { cy.createUser(name, email); // this command returns the wrapped id response referenced below cy.get("@id").as("contactId");});
Next, I discovered Cypress has a great example of using .as() and .then() when Sharing Context so now I had what I needed for the it() block.
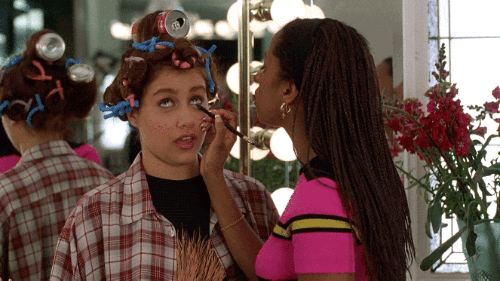
Yeah, it’s happening!
beforeEach(() => { cy.createUser(name, email); cy.get("@id").as("contactId");});afterEach(() => { // I'm not sure yet});describe("Experimenting with aliases", () => { it("A User is created", () => { cy.get("@contactId").then(function () { cy.api({ headers, auth, method: "GET", url: "/url/used/to/test/for/an/existing/user", }).then((response) => { // Expecting the response status code to be 200 expect(response.status).to.eq(200); expect(response.body).contain("users", `${this.contactId}`); }); }); });});
Don’t forget the function!!
Okay, this is good! I’m so close!!

What I didn’t know what that I needed to call the alias again, paired with .then(function () {}) to make the alias accessible in the afterEach()
beforeEach(() => { cy.createUser(name, email); cy.get("@id").as("contactId");});afterEach(() => { cy.get("@contactId").then(function () { cy.deleteContact(this.contactId); });});describe("Experimenting with aliases", () => { it("A User is created", () => { cy.get("@contactId").then(function () { cy.api({ headers, auth, method: "GET", url: "/url/used/to/test/for/an/existing/user", }).then((response) => { // Expecting the response status code to be 200 expect(response.status).to.eq(200); expect(response.body).contain("users", `${this.contactId}`); }); }); });});
Was I able to use this with multiple tests? Absolutely. Is it terrible? I don’t know! Fill me in! In the end, I had DRY-er tests and I learned something valuable.
What’s your experience been with aliases lately?
Till next week…

All my posts are free to read and clicking subscribe will bring each post to your inbox. If my work brings you joy, and you’d like to support it, you can become a paid subscriber by clicking the button above. If a paid subscription is not your thing, you can support my caffeine addiction writing by clicking the button below! Thanks!
Written with “Have a relaxing time with the soothing night sky and meditation music! Also for sleepless nights” playing in the background.
Reply